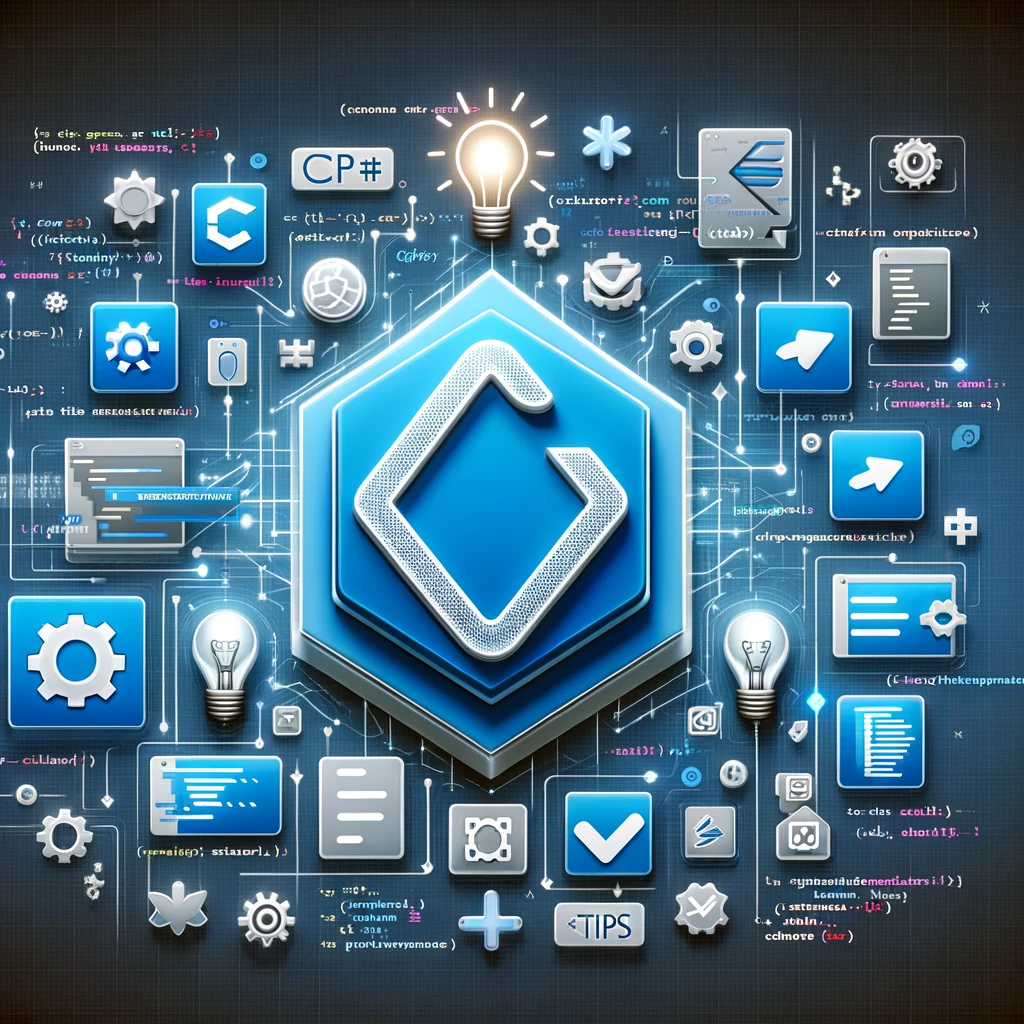
在C# 使用正規表示式(Regular expression)
正規表示式(英語:Regular expression,常簡寫為regex、regexp或RE),又稱規律表達式、正規表達式、正規表示法、規則運算式、常規表示法,是電腦科學概念,用簡單字串來描述、符合文中全部符合指定格式的字串,現在很多文字編輯器都支援用正規表達式搜尋、取代符合指定格式的字串。許多程式設計語言都支援用正規表達式操作字串,如Perl就內建功能強大的正規表達式引擎。正規表達式這概念最初由Unix的工具軟體(例如sed和grep)普及開。來源:https://zh.wikipedia.org/zh-tw/%E6%AD%A3%E5%88%99%E8%A1%A8%E8%BE%BE%E5%BC%8F
在面對有成千隻的程式,要找到某個SQL是相當的煩人的。搭配C#正規表示式,快速將SQL語法取出。正規表示式,在爬網路資料時一樣受用。
// 使用正規表示式 取得 SQL 資料
using System;
using System.Text.RegularExpressions;
class Program
{
static void Main(string[] args)
{
string input = "SELECT * FROM Customers; INSERT INTO Orders (CustomerID, OrderDate) VALUES ('ALFKI', '2022-03-06');";
Regex regex = new Regex(@"(SELECT[\s\S]*?;|INSERT[\s\S]*?;|UPDATE[\s\S]*?;|DELETE[\s\S]*?;)");
MatchCollection matches = regex.Matches(input);
foreach (Match match in matches)
{
if (match.Success)
{
Console.WriteLine("SQL statement: " + match.Value.Trim());
}
}
}
}
搭配先將C/C++註解移除效果更好
// 移除 C/C++ 的註解
using System;
using System.Text.RegularExpressions;
class Program
{
static void Main(string[] args)
{
string input = "/* This is a C-style comment. */ // This is a C# comment.";
Regex regex = new Regex(@"/\*([^*]|[\r\n]|(\*+([^*/]|[\r\n])))*\*+/|//.*");
MatchCollection matches = regex.Matches(input);
foreach (Match match in matches)
{
if (match.Success)
{
Console.WriteLine("Comment: " + match.Value.Trim());
}
}
}
}